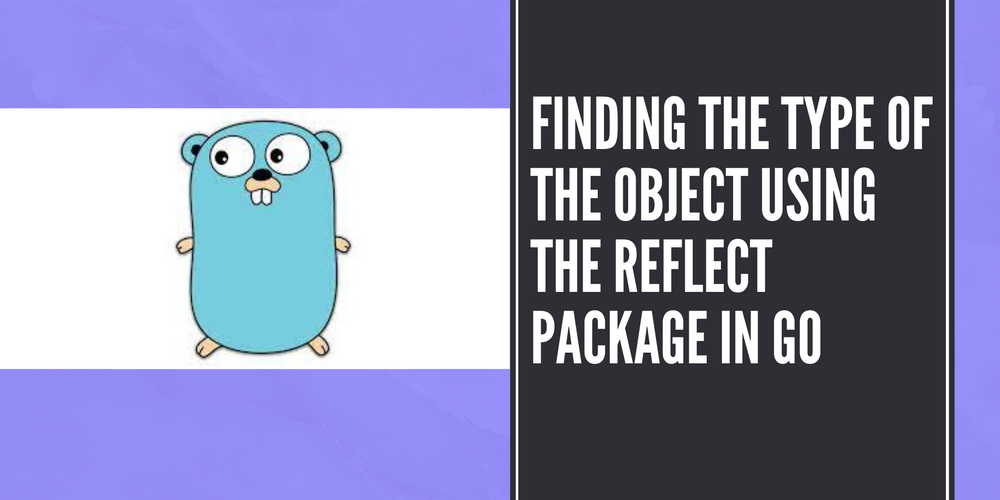
How to find the type of an object in Go
Finding the type of the object using the reflect package in Go-
Sriram Thiagarajan
- September 15, 2021
How to find the type of object with reflect in Go
reflect
package helps us in finding the type of object in Go. It is part of the standard library and so you can just import the package
package main
import (
"fmt"
"reflect"
)
func main() {
isActive := false
name := "First name"
age := 32
score := 34.32
var smallFloat float32 = 2.42
names := []string {"name 1", "name 2"}
fmt.Println("Using Reflect package\n");
fmt.Println(reflect.TypeOf(isActive))
fmt.Println(reflect.TypeOf(name))
fmt.Println(reflect.TypeOf(age))
fmt.Println(reflect.TypeOf(score))
fmt.Println(reflect.TypeOf(smallFloat))
fmt.Println(reflect.TypeOf(names))
fmt.Println("\nUsing String formatting\n");
fmt.Printf("%T\n", isActive)
fmt.Printf("%T\n", name)
fmt.Printf("%T\n", age)
fmt.Printf("%T\n", score)
fmt.Printf("%T\n", smallFloat)
fmt.Printf("%T\n", names)
}
Output
Using Reflect package
bool
string
int
float64
float32
[]string
Using String formatting
bool
string
int
float64
float32
[]string
Summary
This is a quick post on the different ways to figure out the type of object in Go
Stay tuned by subscribing to our mailing list and joining our Discord community