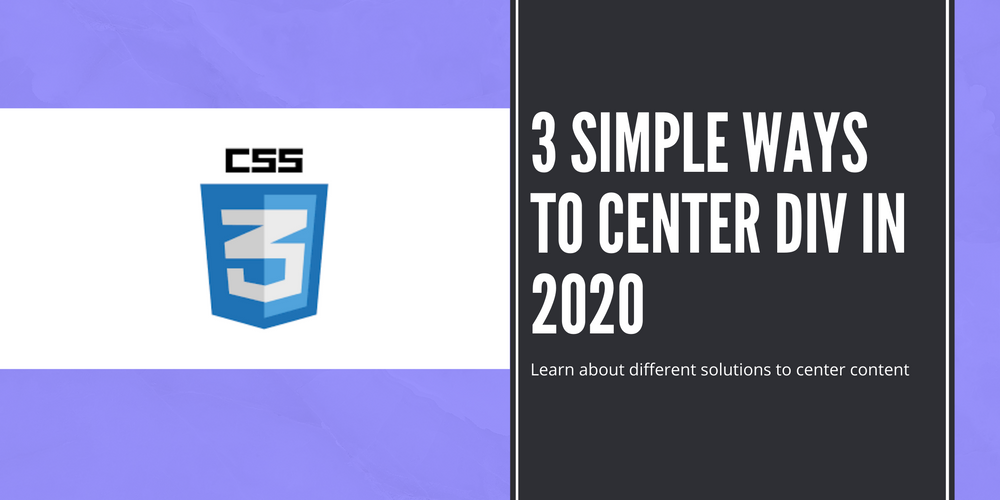
3 Simple ways to center div in 2020
This has been an age old question for frontend dev on how to center a div in a page. As you can imagine, there are multiple clever solutions which developers have used to achieve this.-
Sriram Thiagarajan
- November 1, 2021
3 Simple ways to center div in 2020
This has been an age old question for frontend dev on how to center a div in a page. As you can imagine, there are multiple clever solutions which developers have used to achieve this. As browsers have evolved over the years, we can do things in a simple way as opposed to some tricks which have been used in the past.
TLDR: Use the Flex or Grid solution mentioned below
Define the problem
Most of the times, we would like our content to be vertically centered in the page. This will give a nice look to the content and easy for viewers to read. So let’s create a page which will have two divs - One is the page
div and the other is content
div
I am going to demonstrate this in a .Svelte file but these solutions can be achieved in plain HTML and CSS
Page will cover the whole height of the viewport and so we will give the value 100vh
Content doesn’t need any more styles but we will provide the width, height, background-color to get a better visual representation of the solution.
<style>
.content {
width: 200px;
height: 200px;
background-color: blue;
}
.page {
height: 100vh;
}
</style>
<div class="page">
<div class="content"></div>
</div>
1. Old fashioned Way
Before we get to the solution we can use now. It will help to understand the old but still golden way to center a div.
Mark the element with absolute
positioning and then set the top to 50% and then transform the element to -50%
.content {
position: absolute;
top: 50%;
left: 50%;
transform: translateY(-50%) translateX(-50%);
}
This method can still be used and it is quite good. One major disadvantage is when you want to add other transforms or animations to the content, it might not work as intended
2. Flex solution
Flex box has been a great tool set for frontend dev to achieve various styling needed for the elements. This has been my go-to solution for most of the CSS positioning solutions now and it is really easy to center a div vertically as well.
.page {
display: flex;
align-items: center;
justify-content: center;
}
This solution involves adding styles to the parent container instead of the child content. This will ensure that all the children within the div are aligned properly.
align-items
and justify-content
controls the position of the children based on flex-direction
property. Default value of flex-direction
is row
and so in this case, align-items
control the vertical alignment and justify-content
controls the horizontal alignment.
One other good thing about this solution, is that even if you have more than one children, all of them will be centered based on the available space.
Bonus: You can also add styles to the child element to center it vertically provided that the parent is a flexbox
.page {
display: flex;
}
.content {
align-self: center;
margin: 0 auto;
}
3. Grid solution
Grid on CSS is also very cool technique to create a variety of different layouts. We can use that as well to vertically center a div although it might be a overkill if you just have only one child inside.
Note: The previous solution of setting align-items
and justify-content
also works with display:grid
but we will look into more on how to use grid properly.
.content {
grid-row: 2 / span 1;
margin: 0 auto;
}
.page {
display: grid;
grid-template-columns: 1fr;
grid-template-rows: repeat(3, 1fr);
}
We are dividing the spaces available into multiple pieces, The column will consist of 1fr
which is short for 1 flexible length
. The browser will take all the available space and assign it to each row or column based on how they are split.
Consider the case of grid-template-rows
, we are using the repeat
function to duplicate the 1fr
unit three times. This will result in creating three row and the available space is split equally between them. If you want the second row to have double the space than other, you can use 2fr
instead of 1fr
After dividing the space, we need to assign the content to one of the space in the grid. We can do this by setting the grid-row
property in the content and specifying the start-row and then how many row it should span. Since we have three rows now, the content will start from 2nd row and span for 1 row which mean it will just occupy the 2nd row.
Since we are not dividing the columns into more pieces, you can set the margin: 0 auto
in the child to center the content horizontally. You could also divide the column into three pieces and set the content in the 2nd column and span 1 column as well.
Bonus: You can have the element span multiple rows which can be useful if you want some element to be bigger than others
Conclusion
Although the concept of placing the element in the center might seems very simple, there are multiple solutions to choose from and each has some limitations. You can choose the one which is very trivial to start with and then add complexity if needed. We recommend using the flex for simple usecases and grid solution for more complex use cases. Also remember to check the browser compatibility of these properties to make sure these properties work in your supported browser’s list.
You can check the browser compatibility in this link below