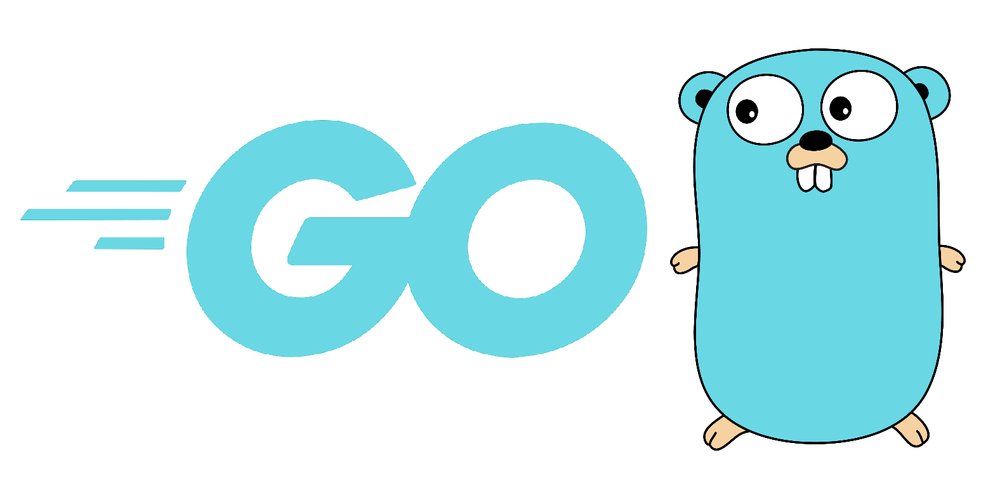
How to create a multiline string in Go
A multiline string can be useful when you want to show the user of your app in a readable format. This article shows you how you can achieve multiline strings in Go-
Sriram Thiagarajan
- August 24, 2021
How to create a multiline string in Go
A multiline string can be useful when you want to show the user of your app in a readable format. This article shows you how you can achieve multiline strings in Go
Strings in Go
Strings in Go is a variable-length of characters where each character is represented by one or more bytes of UTF-8 encoded data. It is read-only and immutable which means that you cannot change the value after it is assigned.
awesomeStr:= "My string";
awesomeStr[0] = 'S'; // Throws error from compiler
Creating multi-line string using raw string literals (Backtick)
We can make use of backtick and have it easy to read and write in code. All the indentation is also respected.
package main
import "fmt"
func main() {
str := `Documentation for the usage of the API
*Param 1* - Useful for feature A
*Param 2*- Useful for feature B
`
fmt.Println(str)
}
Output
Documentation for the usage of the API
*Param 1* - Useful for feature A
*Param 2*- Useful for feature B
For more details on Go string literals - https://golang.org/ref/spec#String_literals
Creating multi-line string with \n characters
You can add \n
character to the string variable to display the items in a new line. This method will work for simple cases but it makes the code messy and hard to read
package main
import "fmt"
func main() {
multiline := "Documentation for the usage of the API\n\n*Param 1* - Useful for feature A\n*Param 2*- Useful for feature B"
fmt.Println(multiline)
}
Output
Documentation for the usage of the API
*Param 1* - Useful for feature A
*Param 2*- Useful for feature B
String interpolation in Go
String interpolation in Go can be achieved using the printf
method from the fmt
package. Here are some examples of String interpolation in Go.
package main
import "fmt"
func main() {
name:= "Student 1"
total:= 426
grade := 'B'
avg := 8.325
fmt.Printf("Name is %s\n", name)
fmt.Printf("Total is %d\n", total)
fmt.Printf("Grade is %c\n", grade)
fmt.Printf("Average is %f\n", avg)
}
Table of string interpolation verb in Golang
Data Type | String interpolation verbs |
---|---|
int | %d |
string | %s |
char | %c |
float | %f |
Try out the string interpolation in Go using the codeshare link
https://www.jdoodle.com/embed/v0/4sKU
Summary
Stay tuned by subscribing to our mailing list and joining our Discord community