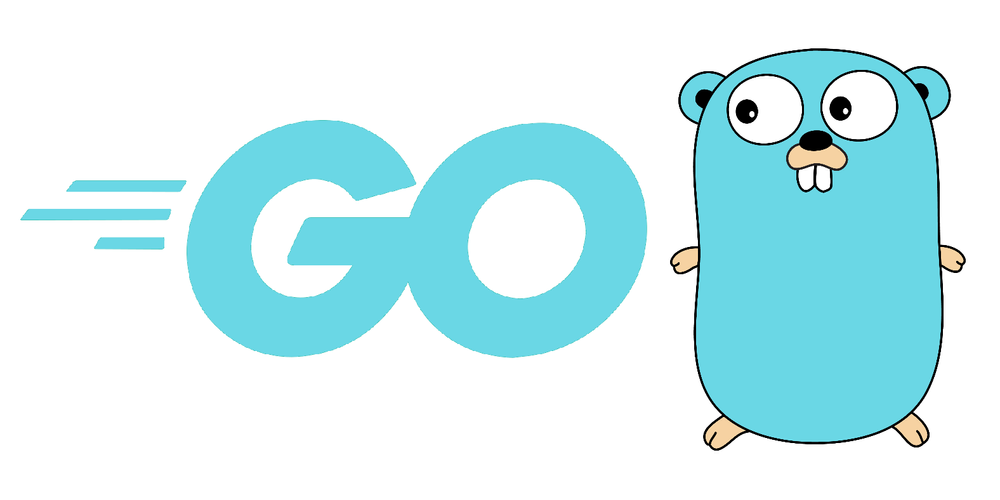
How to concatenate strings in Go
Different ways to concatenate strings in Go with examples-
Sriram Thiagarajan
- July 22, 2021
How to concatenate strings in Go
In this post, we will look at a few ways to concatenate strings in Go
Concatenate using the ’+’ and ’+=’ operator
Most of the time we can use this simple method to concatenate strings and not worry about performance issues. Just use the ’+’ operator
firstname := "Stephen"
lastname := "Hawking"
fullname := firstname + " " + lastname
fmt.Println(fullname)
Concatenate using ’+=’ operator
a := "testing"
b := " is essential"
a += b
fmt.Println(a)
When performing the concatenation operation inside a loop and when performance is important, you can use the below efficient method
Concatenate using String builder
We can use the strings package to perform efficient concatenation on strings. WriteString
function can be used to add strings to the variable.
{var}.String()
function is used to get the data in string type
package main
import (
"fmt"
"strings"
)
func main() {
var sb strings.Builder
for i := 0; i < 100; i++ {
sb.WriteString(" Appending inside loop")
}
fmt.Println(sb.String())
}
-
Import the
strings
package which provides the strings.Builder type -
Create a variable of
strings.Builder
type. This will initialize the variable to Zero-Value of that type which is an empty string -
Appending the data to the string can be done using the
WriteString
function -
When you want the data of the variable in string type, call the
String()
function
Summary
Simple concatenation can be done using the ’+’ operator and for a more efficient method using the “strings” package