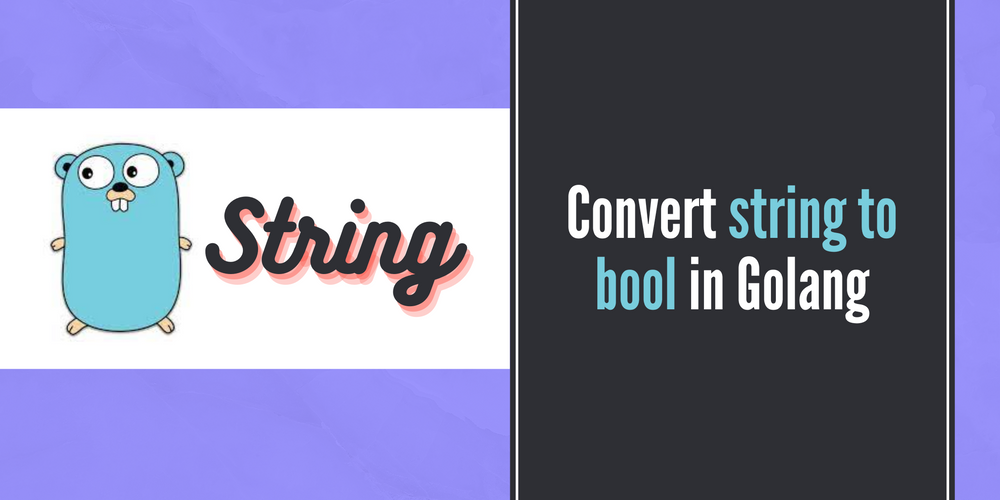
Convert string to bool in Golang
Converting string to bool can be done using the strconv.ParseBool method. It tries to return a boolean value from a string. It accepts the strings in the following format 1, t, T, TRUE, true, True, 0, f, F, FALSE, false, False-
Sriram Thiagarajan
- October 29, 2022
Convert string to bool in Golang
Converting string to bool can be done using the strconv.ParseBool
method. It tries to return a boolean value from a string. It accepts the strings in the following format
// True string values
1, t, T, TRUE, true, True
// False string values
0, f, F, FALSE, false, False
Anything else apart from these value will throw an error when using the strconv.ParseBool
method.
// stringVal can be set to any one of the above valid values.
value, err := strconv.ParseBool(stringVal)
Example for converting string to bool in Golang
package main
import (
"fmt"
"strconv"
)
func main() {
// Success conversion with no error
boolString := "True"
boolVal, err := strconv.ParseBool(boolString)
if err != nil {
fmt.Println("Error in conversion", err)
} else {
fmt.Println("Converted Boolean value - ", boolVal)
}
// Error conversion
boolErrString := "Abcd"
boolErrVal, err := strconv.ParseBool(boolErrString)
if err != nil {
fmt.Println("Error in conversion", err)
} else {
fmt.Println("Converted Boolean value - ", boolErrVal)
}
}
Steps to convert
- Create a variable of string which will contain one of the valid formats of representing bool as string
- Pass the value to
strconv.ParseBool
method to get a value or error - Check the error variable is there is any error and if so print the error
- If there is no error, you can use the value
You can check the official documentation for more details - https://pkg.go.dev/strconv#ParseBool