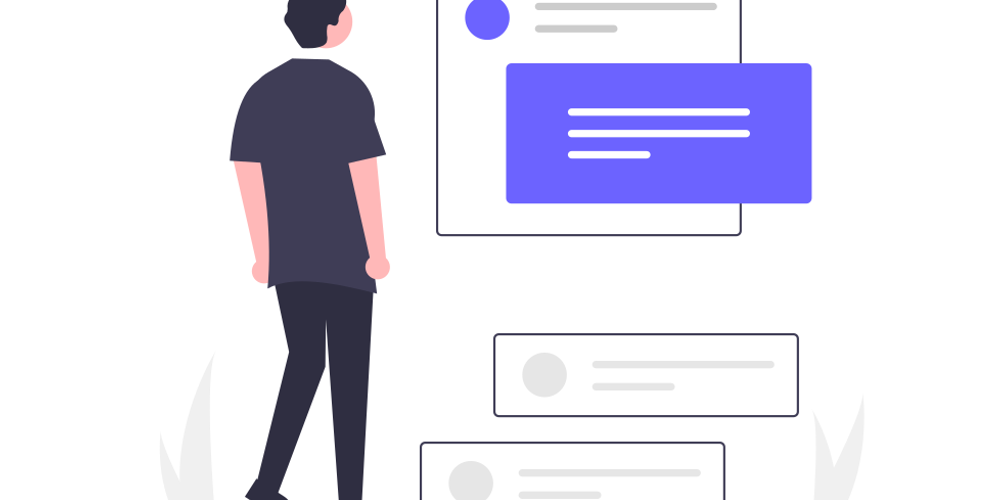
Async and Await - A Beginner's Guide to JavaScript Concurrency Features
Async and Await - A Beginner's Guide to JavaScript Concurrency Features-
Sriram Thiagarajan
- August 5, 2023
Async and Await: A Beginner's Guide to JavaScript Concurrency Features
Ever found yourself in a position where you're trying to make a nice cup of coffee, but your kettle takes forever to boil? And while you're waiting, you can't do anything else because you need to watch the boiling kettle? Doesn't it grind your gears? Well, welcome to the world of synchronous programming.
"Synchronous programming is like a boiling kettle, you can't do anything else until it's done."
Fortunately, in the realm of JavaScript, developers have a more practical approach - enter asynchronous programming. Asynchronous programming, or as us web wizards like to call it, Async, allows you to perform other tasks while waiting for that metaphorical kettle to boil.
- So, why is asynchronous programming useful in web development?
- Performance: In the synchronous world, your website would become unresponsive until all tasks are complete. However, with asynchronous programming, tasks are executed in the background allowing your website to remain interactive. This leads to a better user experience.
- Efficiency: Asynchronous programming allows multiple tasks to be managed concurrently, meaning your application can perform more tasks in the same amount of time.
In this article, we'll deep dive into the concept of async and await in modern JavaScript. So, buckle up and get ready for some asynchronous awesomeness!
Understanding JavaScript Promises
Async/await is built on top of Promises. So let's first understand Promises in JavaScript.
Promises in JavaScript are objects representing the eventual completion or failure of an asynchronous operation. They're like IOUs from the JavaScript engine. "I owe you one result, coming soon!"
A Promise is in one of these states:
- pending: The Promise's outcome hasn't yet been determined, because the asynchronous operation that will produce its result hasn't completed yet.
- fulfilled: The asynchronous operation has completed, and the Promise's result is a value.
- rejected: The asynchronous operation failed, and the Promise's reason is an indication of failure.
Promises come with built-in error handling. With synchronous code, we use try-catch blocks for error handling. Asynchronous operations with callbacks don't have a defined mechanism for handling errors. Earlier, developers would resort to the infamous "callback hell" or "pyramid of doom". Promises rescue us from this doom with their error handling capabilities.
To handle error in promises, we use the .catch() method. The .catch() method returns a Promise and deals with rejected cases only. It works the same as calling .then(undefined, onRejected). Here is a simple example:
Code | Description |
---|---|
Promise.resolve("success").then(function (data) {// handle success case}); |
This code creates a Promise that is immediately resolves with the data "success". The resulting Promise object is then handled with a .then() block, which will execute its callback function given the Promise was resolved. |
Promise.reject("failed").catch(function(reason){
// handle error here
});
|
This code creates a Promise that is immediately rejected with the reason "failed". The resulting Promise object is then handled with a .catch() block, which will execute its callback function given the Promise was rejected. |
Async_method().then(function (data) { // Handle the success event. Use the data to do some calculation}).catch(function (reason) { // Handle the error case to show some pop up that something when wrong}); | This is an typical example of how the Async methods are handled in JavaScript with Promises with consisting of both the success and error callback function which will handle the respective cases. |
Thus, Promises not only improve the readability of asynchronous code but also provide a robust error handling mechanism, making life easier for JavaScript developers around the globe.
Working with Callback Functions and How to Avoid Callback Hell
Callback Hell is a phenomenon that occurs when you chain too many asynchronous operations together, leading to code that's nearly impossible to read and debug. It's also affectionately known as the Pyramid of Doom, and it looks something like this:
function requestData(callback) {
networkRequest(function(response1) {
anotherNetworkRequest(response1, function(response2) {
yetAnotherNetworkRequest(response2, function(response3) {
callback(response3);
});
});
});
}
Feel your brain tying itself into knots? That's the feeling of descending into Callback Hell. But fear not, for salvation is at hand. And, it comes in the form of Promises.
From Callback Hell to Promise Heaven
Promises were introduced to mitigate the issues of Callback Hell, making asynchronous code easier to write and understand. When you chain multiple promises, it can still lead to code that's hard to read and understand. Let's consider an example:
requestData().then(function(response1) {
return anotherRequest(response1).then(function(response2) {
return yetAnotherRequest(response2).then(function(response3) {
return finalRequest(response3);
})
})
})
Though it's a step up from our Callback Hell, it's still not the most readable and maintainable. This is where our superheroes - Async/await can help avoid callback hell.
Async/ Await - What are they and Why do You Need Them?
Async/await was introduced in ES2017, riding to our rescue to combat the nesting issue, colloquially known as "callback hell," and the sometimes confusing behavior of promises. These two words are like superheroes in the JavaScript world, making asynchronous programming much more straightforward and readable.
Hint: Asynchronous operations are operations that can happen independently of the main program flow.
Let’s delve further into how exactly they simplify our code:
- The async keyword turns a function into an Async Function, which wraps its return value into a promise. This means, even if you return a non-promise value from an async function, JavaScript wraps it in a resolved promise.
- The await keyword can only be used inside an Async Function and makes JavaScript wait until that promise settles and returns its result.
Now, let's give you a comparative perspective on how async/await trounces the traditional promise syntax:
Promise Syntax | Async/Await Syntax |
---|---|
function foo() { return Promise.resolve('Hello'); } foo().then(alert); |
async function foo() { let result = await Promise.resolve('Hello'); alert(result); } foo(); |
As you can see, the async/await syntax is more intuitive and easy to comprehend. It's like going from reading a complex novel to a children's storybook. However, remember that with great power comes great responsibility; while async/await simplifies asynchronous operations, it's crucial to handle errors properly to avoid turning our JavaScript wonderland into a chaos-filled jungle.
Remember:
If you don't catch an error in an async function with a try/catch block, it will result in an unhandled promise rejection, which is a serious issue in JavaScript.
So, as you're venturing further into the depths of JavaScript, remember to use the async/await syntax wisely. It's not just a tool, but your ally in writing clean, understandable, and maintainable code.
Handling Errors Gracefully with Async and Await
Async and Await, come with a built-in mechanism for handling errors: the try-catch block.
Remember: A good developer is like a skilled fencer, always on guard and ready to parry any incoming errors.
Here's a simple example of how you can employ a try-catch block within an async function:
async function fetchJesterJokes() {
try {
let response = await fetch('https://api.jesterjokes.com/jokes');
let data = await response.json();
console.log(data);
} catch (error) {
console.log('Alas, the jester has stumbled! ', error);
}
}
The try section is where your normal code execution will take place, while the catch section is your safety net, ready to catch any errors that might occur.
- Try: Here lies the code that may potentially throw an error. It's like a medieval jousting event, but instead of knights, we have lines of code, and instead of lances, we have... well, more lines of code.
- Catch: If an error does occur in the try block, execution will immediately jump to the catch block, saving your program from a gruesome fate. It's like the net beneath a trapeze artist, always ready to catch them if they fall.
Remember, fellow knight of the code realm, error handling in JavaScript is a crucial part of your toolkit. Just as a castle requires a sturdy defense, so too does your code. With Async, Await and Try-Catch, you'll be well-equipped to face any challenge that comes your way.
Common mistakes when using that Async/Await syntax
-
- Remember to include 'await' when calling asynchronous functions to avoid undefined results or unresolved Promises.
- Ensure proper error handling in async/await usage by wrapping code in try-catch blocks.
- Avoid using async/await in synchronous contexts
- Be mindful not to overuse async/await as it could lead to performance issues. Other patterns like Promises or callbacks may be more efficient for simpler operations.
Debugging Async and Await Code
Let's dive deep into the mysterious ocean of debugging Async/Await in JavaScript. Debugging asynchronous code can sometimes feel like trying to find your way in a pitch-black room with a tiny flashlight. However, fear not, for we shall shed some light on the different ways you can debug Async/Await code and transform that room into a well-lit stadium.
One of the common techniques is console logging. It's like leaving breadcrumbs in a forest to track your path. But instead of breadcrumbs, you leave logs, and instead of a forest, you have your code. Sounds less adventurous, but it's quite effective.
"console.log()" is the flashlight of JavaScript debugging. It might not be the most elegant solution, but it sure gets the job done."
However, we have other sophisticated methods apart from console logging. Let's take a look at them:
- Using DevTools: DevTools in browsers like Chrome and Firefox are like the Swiss Army knife for developers. You can use breakpoints, step through the code, inspect variables, and much more. It's like having a magnifying glass to inspect every detail of your code.
- try...catch blocks: This is a great way to handle exceptions in your Async/Await code. You can also log any errors that occur during the execution of your asynchronous code. This method is like having a safety net while walking on a tightrope.
- Using a linter: A linter like ESLint can help you spot errors before they become a problem. It's like having a fortune teller who warns you about future problems.
Let's summarize these methods in our debugging toolkit:
Method | Description |
---|---|
Console logging | Leaving logs in your code to track execution |
DevTools | Using the development tools provided by the browser |
try...catch blocks | Handling exceptions and logging errors |
Using a linter | Checking code for potential errors before execution |
So there you have it, brave explorer. With these tools at your disposal, debugging Async/Await code should no longer feel like navigating a dark labyrinth. Now, go forth and debug with confidence!
Real-World Examples of Async and Await in Action
If you want to learn how to use the async/ await syntax to make an API call and handle error in Svelte application, you can have a look into this article where we explains that in details
https://eternaldev.com/blog/how-to-make-an-api-call-in-svelte
In Conclusion
Let's wrap up our jaunt through the forest of modern JavaScript. We ventured deep into the undergrowth, discovering the exhilarating possibilities of Callbacks, Promises, and Async/Await syntax.
Callbacks
In the context of our exploration, we first encountered Callbacks. As the elder of the group, Callbacks seem quite humble. Yet, don't be fooled by their simplicity. They're foundational to JavaScript and essential to understanding how to manage asynchronous operations. Remember, a Callback is nothing more than a function invoked after the completion of a certain task.
Promises
We then encountered Promises. As the name suggests, they promised a cleaner way to handle asynchronous operations. They represented an evolution, providing us with a more powerful tool to handle the unpredictable nature of asynchronous tasks. With their resolve, reject, and finally methods, they offered a more structured way to approach the wilds of async code.
Async/Await
Finally, we met the most modern tool in our arsenal, Async/Await. It's like a machete that cuts through the tangled vines of promise chains. With Async/Await, we can write asynchronous code that looks and behaves as if it's synchronous. It's a game-changer, providing a clear path and making the whole journey through asynchronous operations decidedly more comfortable.
Technique | Pros | Cons |
---|---|---|
Callbacks | Simple and foundational. | Can lead to "callback hell" if not managed properly. |
Promises | Structured error handling, chaining. | Complexity increases with nested promises. |
Async/Await | Synchronous-like code, improved readability. | Error handling can be tricky. |
Remember, the choice between Callbacks, Promises, and Async/Await doesn't boil down to which is 'the best'. It's contextual, depending on your specific project requirements, your team's familiarity with the concepts, and the nature of the asynchronous operations you're dealing with in your JavaScript application.
Keep exploring, keep learning, and don't stop coding!